The Timer1 module is a 16-bit timer/counter within most PIC MCU devices. Timer1 can increment up to a value of 65535 before it overflows back to zero. Because the timer is built into an 8-bit device, the 16-bit timer register is broken into two 8-bit registers (TMR1L and TMR1H) and increments in a similar way to an 8-bit timer with 8-bit prescaler. Timer1 has other built-in features that make it very useful for many different applications, such as the following:
- 16-bit timer/counter register pair (TMR1H:TMR1L)
- Programmable internal or external clock source
- Prescaler
- Interrupt on overflow
- Wake-up on overflow (external clock, Asynchronous mode only)
The Timer1 module has 2 distinct modes in which it can operate:
Timer Mode: In Timer mode, Timer1 functions as a timer. It increments its value at a specified rate based on the clock source it’s configured to use. In this mode, the input clock to the timer is Fosc/4 Once Timer1 reaches its maximum value (which depends on the number of bits it has), it overflows and generates an interrupt (or trigger an action, depending on the configuration). This overflow can be used to measure time intervals, generate periodic events, or trigger specific actions after a certain period.
Counter Mode: In Counter mode, Timer1 functions as an external event counter. It counts the number of external pulses received on its input pin (usually RC1/T1OSI/CCP2 or RC0/T1OSO/T1CKI) and increments its value accordingly. This mode is often used for applications where you want to count external events such as pulses from an external sensor, encoder, or any other source that generates discrete events.
I refer to the overview of Timers, Timer0 and Timer2 for their respective information.
1. Timer Mode
We will focus on the timer mode, even though the other mode might be just as interesting for certain applications. However, I will briefly mention how to configure the counter mode as well thruoghout the text. The other three important registers are:
Registers | Description |
T1CON | This register is used to configure Timer 1’s prescaler, clock source, etc. |
TMR1L | This registers hold the lower 2 digits of the counter, from which the timer starts counting toward its maximum value. |
TMR1H | This registers hold the higher 2 digits of the counter, from which the timer starts counting toward its maximum value. |

T1CKPS1:T1CKPS0:Timer1 Input Clock Prescale Select bits
11 = 1:8 prescale value
10 = 1:4 prescale value
01 = 1:2 prescale value
00 = 1:1 prescale value
T1OSCEN: Timer1 Oscillator Enable Control bit
1-Oscillator is enabled
0-Oscillator is shut-off (the oscillator inverter is turned off to eliminate power drain)
T1SYNC: N/A for this timer in timer mode.de
TMR1CS: Timer1 Clock Source Select bit
1-External clock from pin RC0/T1OSO/T1CKI (on the rising edge) – Don’t confuse this with Crystal oscillator on CLKIN/CLKOUT pins
0-Internal clock (FOSC/4)
TMR1ON: Timer1 On bit
1-Enables Timer1
0-Stops Timer1
The prescaler is not as large as for Timer0 or Timer2, with 2 bits; it has a max value of 1:8. To enable and configure Timer 1, the remaining bits have to be set correctly. T1OSCEN = 1; Furthermore, to enable the internal clock (which could be the crystal oscillator/4), the follow bit has to be set: TMR1CS = 0; Finally, the timer1 has to be enabled through the TMR1ON = 0 bit.
1.1 TMR1H & TMR1L Registers
The other important part of the timer mode is to set the timer to the correct delay that you want it to be. This is best explained in my overview tutorial on Timers. Nonetheless, I will give a short recap here as well. Timer 1 requires you to make a Start number, from which it counts towards its maximum where it overflows. This start number can be calculated using the formula described below. In the case of Timer1, which is a 16-bit timer, the starting number will most likely be 2 bytes (or a 4-hexadecimal number), which needs to be split up in 2 separate registers, TMR1H (for the higher byte) and TMR1L (for the lower byte). Again, in my tutorial on timers, I provide a detailed example on exactly how to calculate it.
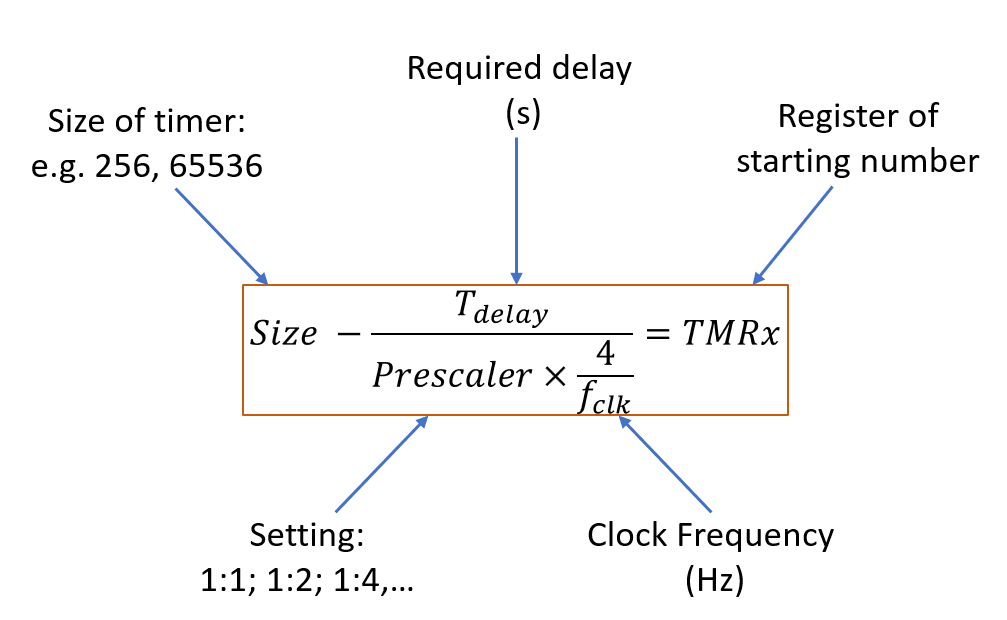
2. Timer Interrupt
To enable the interrupt, we need to enable the global and peripheral interrupts in the INTCON register. This is done by setting both GIE = 1 and PEIE = 1.

Lastly, the TMR1 overflow flag enabler is situated in the PIE1 register and needs to be set to TMR1IE = 1; The TMR1 overflow flag itself is situated in the PIR1 register, and has to be cleared every time an interrupt happened. This can be done by setting TMR1IF = 0;
3. Programming code in MPLAB
Assume we have a PIC16F877A microcontroller with a 20 MHz crystal oscillator. We want to create a timer of 100 ms using Timer1. With the calculations from my tutorial, we can find that the prescaler is set to 8, and the TMR1 starts at 3036. In hexadecimals that is 0x0BDC. Hence, 0x0B goes into TMR1H, while 0xDC goes into TMR1L
#include
#include
#define XTAL_FREQ 20000000 // 20MHz external crystal oscillator frequency
// Configuration bits
#pragma config FOSC = HS // High-Speed Crystal oscillator
#pragma config WDTE = OFF // Watchdog Timer disabled
#pragma config PWRTE = OFF // Power-up Timer disabled
#pragma config BOREN = OFF // Brown-out Reset disabled
#pragma config LVP = OFF // Low-Voltage Programming disabled
#pragma config CPD = OFF // Data memory code protection off
#pragma config WRT = OFF // Flash Program Memory Write protection off
#pragma config CP = OFF // Flash Program Memory Code protection off
// Function prototype
void interrupt timer1_isr();
void main()
{
TRISDbits.TRISD0 = 0; // Configure RD0 (LED) as output
// Timer1 - T1CON Register Configuration
T1CONbits.T1CKPS1 = 1; // Set prescaler to 1:8
T1CONbits.T1CKPS0 = 1;
T1CONbits.TMR1CS = 0; // Use internal clock (Fosc/4)
T1CONbits.TMR1ON = 1; // Start Timer1
// TimerCounter register
TMR1H = 0x0B; // Load the time value (0x0BDC) for 100ms delay
TMR1L = 0xDC;
//INTCON Register & PIE1 Register configuration
TMR1IE = 1; // Enable Timer1 overflow interrupt bit in PIE1 register
GIE = 1; // Enable Global Interrupt
PEIE = 1; // Enable the Peripheral Interrupt
while (1)
{
// No need to toggle PORTD; only control RD1
}
}
// Timer1 interrupt service routine
void interrupt timer_isr()
{
if (TMR1IF == 1)
{
RD0 = !RD0; // Toggle RD0 (LED) state
TMR1H = 0x0B; // Load the timer value for the next 100ms delay
TMR1L = 0xDC;
TMR1IF = 0; // Clear Timer1 interrupt flag in PIR1 register
}
}